Contract Programming
Contracts are a breakthrough technique to reduce the programming effort for large projects. Contracts are the concept of preconditions, postconditions, errors, and invariants. Contracts can be done in C++ without modification to the language, but the result is clumsy and inconsistent.Building contract support into the language makes for:
- a consistent look and feel for the contracts
- tool support
- it's possible the compiler can generate better code using information gathered from the contracts
- easier management and enforcement of contracts
- handling of contract inheritance
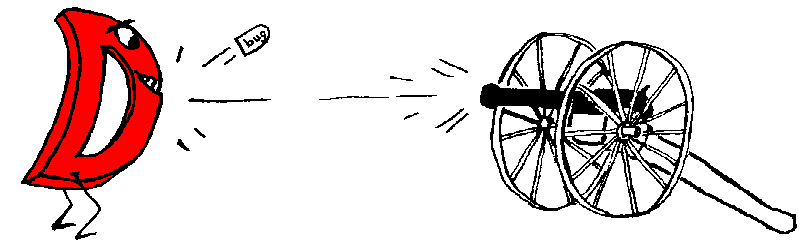
Assert Contract
The most basic contract is the assert. An assert inserts a checkable expression into the code, and that expression must evaluate to true:assert(expression);
C programmers will find it familiar. Unlike C, however, an assert
in function bodies
works by throwing an AssertError
,
which can be caught and handled. Catching the contract violation is useful
when the code must deal with errant uses by other code, when it must be
failure proof, and as a useful tool for debugging.
Pre and Post Contracts
The pre contracts specify the preconditions before a statement is executed. The most typical use of this would be in validating the parameters to a function. The post contracts validate the result of the statement. The most typical use of this would be in validating the return value of a function and of any side effects it has. The syntax is:in
{
...contract preconditions...
}
out (result)
{
...contract postconditions...
}
body
{
...code...
}
By definition, if a pre contract fails, then the body received bad
parameters.
An AssertError is thrown. If a post contract fails,
then there is a bug in the body. An AssertError is thrown.
Either the in
or the out
clause can be omitted.
If the out
clause is for a function
body, the variable result
is declared and assigned the return
value of the function.
For example, let's implement a square root function:
long square_root(long x)
in
{
assert(x >= 0);
}
out (result)
{
assert((result * result) <= x && (result+1) * (result+1) >= x);
}
body
{
return cast(long)std.math.sqrt(cast(real)x);
}
The assert's in the in and out bodies are called contracts.
Any other D
statement or expression is allowed in the bodies, but it is important
to ensure that the
code has no side effects, and that the release version of the code
will not depend on any effects of the code.
For a release build of the code, the in and out code is not
inserted.
If the function returns a void, there is no result, and so there can be no result declaration in the out clause. In that case, use:
void func()
out
{
...contracts...
}
body
{
...
}
In an out statement, result is initialized and set to the
return value of the function.
In, Out and Inheritance
If a function in a derived class overrides a function in its super class, then only one of the in contracts of the function and its base functions must be satisfied. Overriding functions then becomes a process of loosening the in contracts.
A function without an in contract means that any values of the function parameters are allowed. This implies that if any function in an inheritance hierarchy has no in contract, then in contracts on functions overriding it have no useful effect.
Conversely, all of the out contracts needs to be satisfied, so overriding functions becomes a processes of tightening the out contracts.
Class Invariants
Class invariants are used to specify characteristics of a class that always must be true (except while executing a member function). They are described in Classes.
References
Contracts Reading ListAdding Contracts to Java